Activity Lifecycle in Android
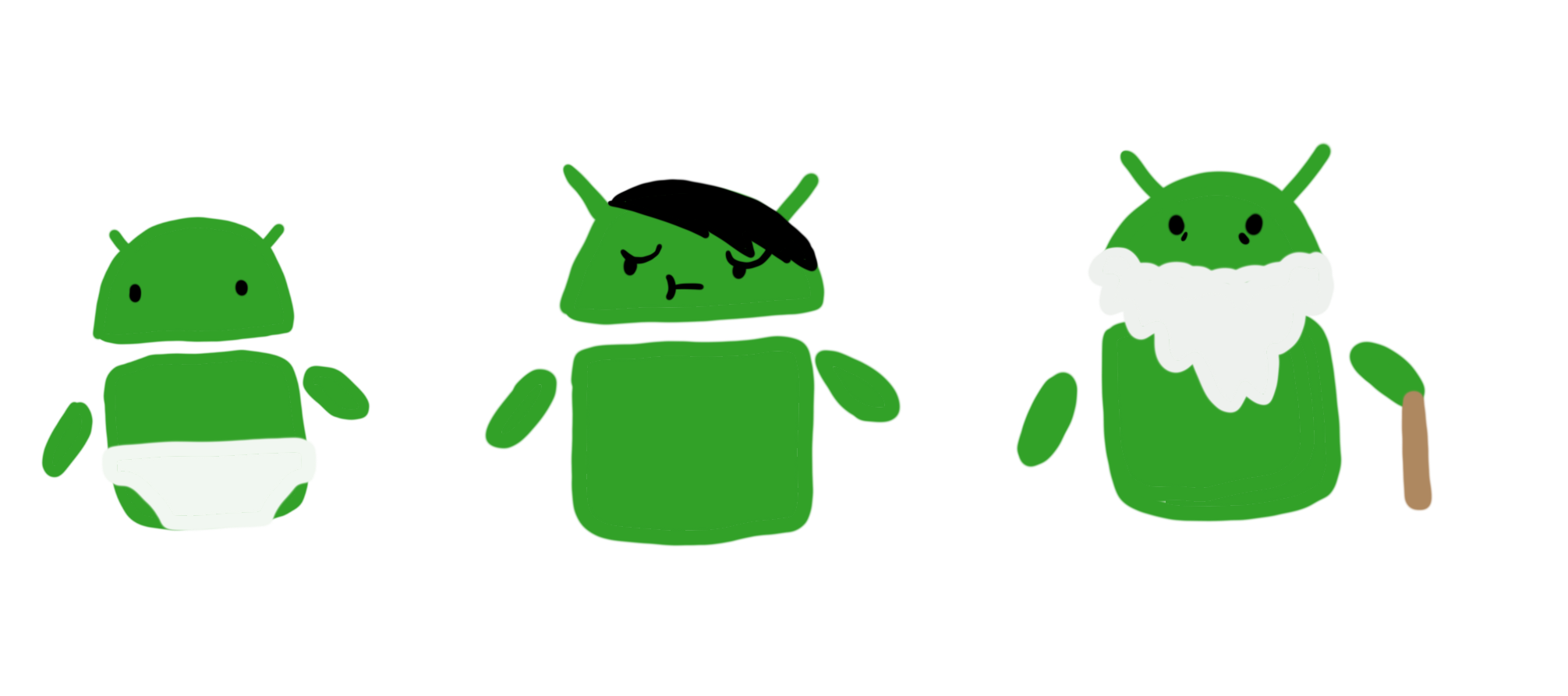
Like everything in this world, activities have lifecycles. Certain methods are called when an event happens. For example, if an activity is in the foreground and the phone starts ringing, that activity is backgrounded. When this happens, onPause
is called. Once the activity is brought back into the foreground onResume
is called. There are seven methods to know about:
- onCreate
- onStart
- onRestart
- onResume
- onPause
- onStop
- onDestroy
You can picture the lifecycle as so:
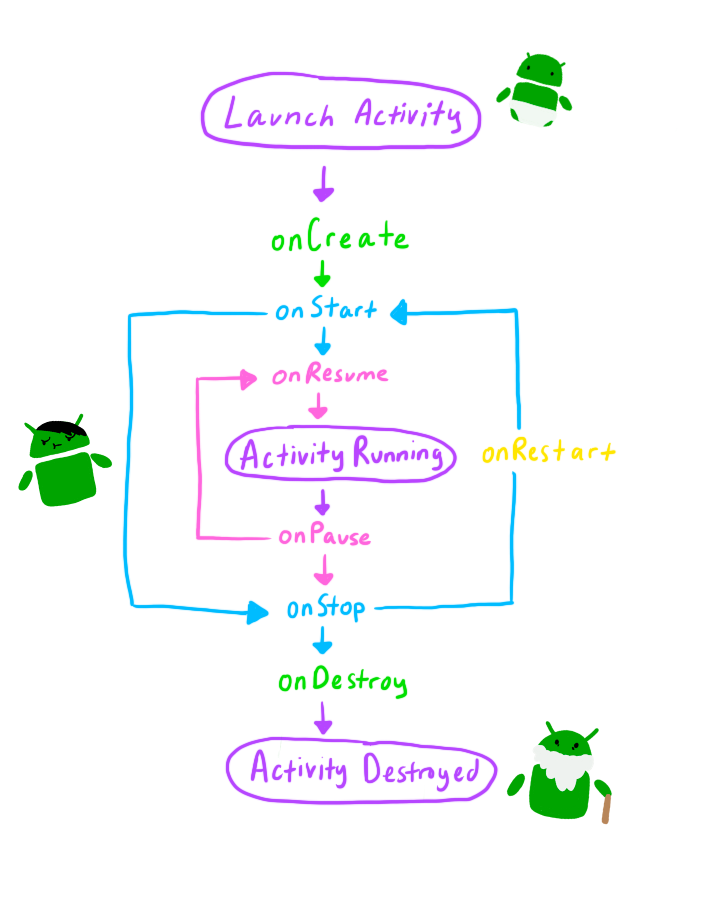
Below is a quick overview of the state of an activity when the methods are called. For more details, refer to the Google developer docs.
- onCreate: when the activity is first created
- onRestart: when the activity has stopped and is about to be created again
- onStart: when the activity was stopped and is being displayed again
- onResume: when the activity is brought back to the foreground
- onPause: when the current activity is leaving the foreground. This operation is quick and you shouldn't perform lengthy transactions in this method
- onStop: when an activity is no longer visible
- onDestroy: before an activity is destroyed
You can override the above methods to have your app perform some kind of action when the event occurs.
override fun onStop() {
super.onStop()
// DO SOMETHING
}
Take care in the methods you override
Thinking I wanted to be extra safe in saving data and making sure the data is always up to date, I overrode these methods too much.
In the Punch Buggy App we read from the Shared Preferences file to get the game information.
We overrode the method onResume
to read from this file. However, because we already read from the file in onCreate
, the data would get out of sync and be messed up because of the order in which the methods were called.
After drawing it out with Beep, we decided to remove the read from the Shared Preferences file from onResume
, and since then everything has been good!