Intents In Android
A quick overview of what intents are in Android
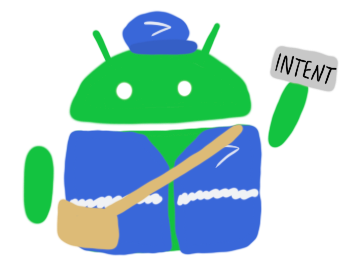
Before going over intents, it's important to know what activities are. In Android, an activity is a window inside of the app you can interact with. Think of a homepage and settings page. Two different views that let you do two different things.
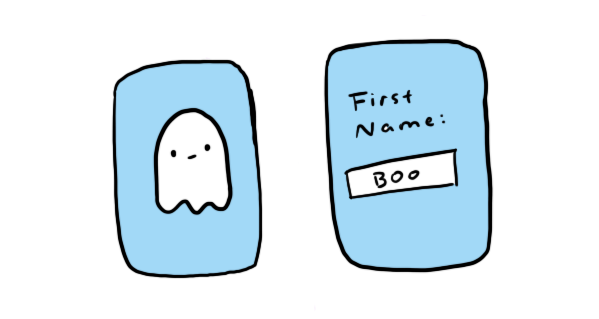
Intents
Intents let you trigger other activities and pass information from one activity to another.
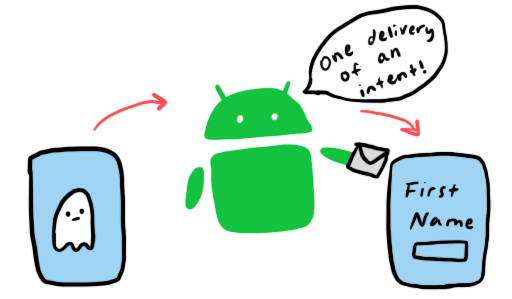
Create an Intent
To do this, you can create an Intent
object. When you create an Intent object, you provide the calling class and the target class it should trigger.
Java:
Intent intent = new Intent(this, target.class);
Kotlin:
val intent = Intent(this, TargetClass::class.java)
Pass data from one activity to another with intents
Next, you can attach information to the intent with putExtra
.
Java:
String user = "boo";
intent.putExtra("firstName", user);
Kotlin:
String user = "boo"
val intent = Intent(this, TargetClass::class.java).apply {
putExtra(firstName, user)
}
Now intent
will have the string boo
saved and mapped to firstName
.
Start the activity
At this point we have an intent object with the first name of the user, boo
. To actually start the target activity, we need to make a call to startActivity
which takes in an intent
object.
startActivity(intent);
Receive an intent
Inside of the class the intent was targeting, you need to get the intent with getIntent()
.
Intent intent = getIntent();
Get the data from the intent
We know that string called firstName
variable should be passed through the intent. To fetch that, we can call getStringExtra
.
String firstName = intent.getStringExtra("firstName");
We have successfully passed the first name of the user from one activity to another using intents.

Next, we will learn about implicit and explicit intents!
This was only a high level overview of intents. The book Head First Android Development is where I learned about intents, and would recommend reading it for a more detailed explanation and actual code examples.